Welcome to my blog! I am Aathil Ducky, and today I will show you how to build an Image to WebP converter micro SaaS using Python Flask. This guide will help you create a tool to optimize images and improve website speed by converting them into the WebP format, a highly efficient image format.
I write content for my website, but I don’t have any idea about what I want to write. At that time, I thought that I am going to create a lot of micro SaaS applications and then share the source code. Maybe those projects will help someone.
For example, anyone can launch the website publicly, and then they can generate passive income through AdSense, charge for the services, or find other ways to monetize it.
Contents
- What is micro Saas application?
- What is my Micro Saas idea?
- how its works (flow diagram)
- Saas app how looks like
- Compare image after compress Webp
- Why image size is importent for webapplication
- Lets go to Code
what is micro Saas application?
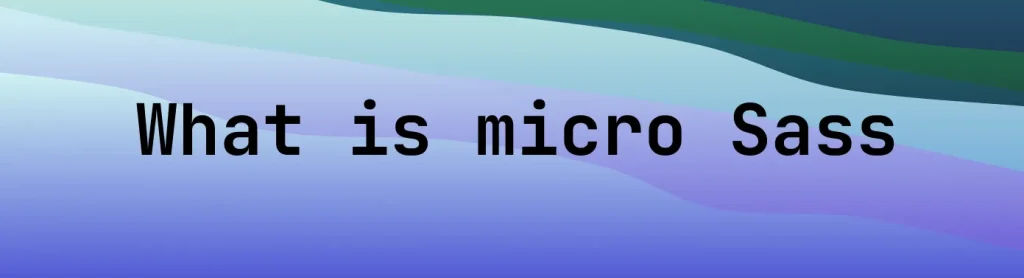
micro saas is a small software tool that help to solve a particular spesific small problems, that helps very spesific group of people that called micro saas.
- micro – this micro means small or focus on one task
- saas – software as a service , we can access in online
for example : – online background remover, noice canceling , auto caption generation and more.
What is my micro Saas idea?
i decited to do IMG to WebP to format. this is my first micro Saas project idea. i wonna build this like a webapplication. using python Flask and html, css, js.
- why i choose this for build micro saas application?
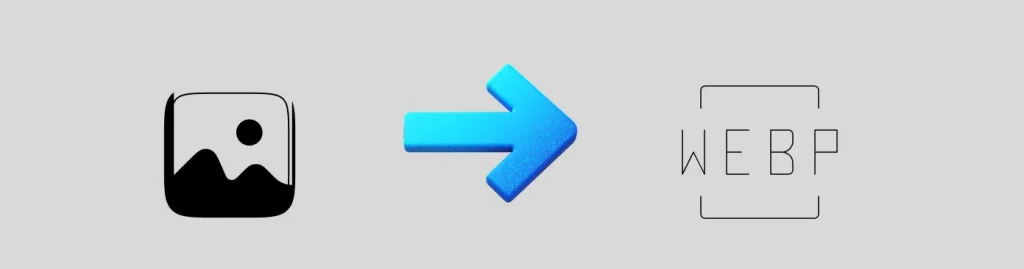
for example consider about me, i am content writer, i want to upload images in webapplication, but some picture size are very high, so it can loadd faster in websites, so that help to improve SEO and user experience.
but, when convert high quality image into webp format , image size will reduces without reducing the quality, its provide better compression then other JPG, PNG like that.
and its suppport lossy and lossless for compression, thats why i choose to build this as a first simple micro Saas application.
Saas app how looks like
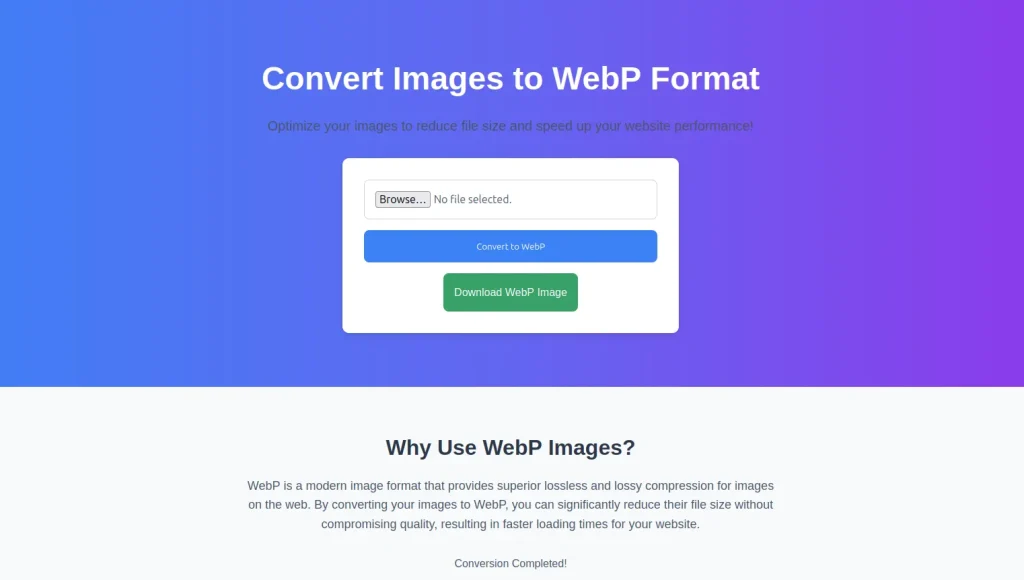
this saas application i created, after selecting the image then we need to press “Convert to WebP” button, after that just download the webp image.
i will explain how i build this webapplication step by step with source code. and finaly i will add this in my github repository, if is it usefull put a star on github repository.
How its works (simple flow diagram)
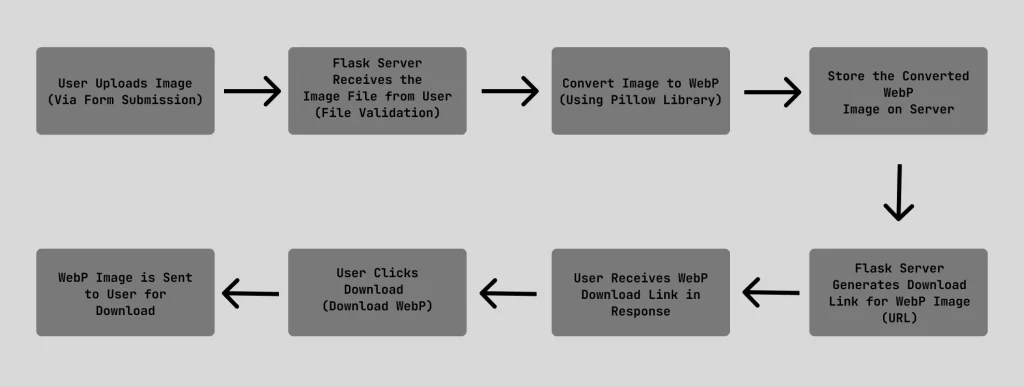
its just simple works flow diagram, this not perfect diagram for this , i did this for showing functionalities of this appication, i wiill explain one by one
- User Uploads Image: The user uploads an image using the webapplication form
- Flask Server Receives the Image: The server processes the uploaded image and validates it. (its only allow given image formats only)
- Convert Image to WebP: use Pillow library to convert the image to WebP format.
- Store Converted Image: The converted WebP image is stored on the server.
- Generate Download Link: generate a URL that can be user download the converted image.
- User Clicks Download: when user click the download link WebP image will download.
- Image Sent to User: The Flask app sends the WebP image to the user for downloads folder.
Compare image after compress Webp
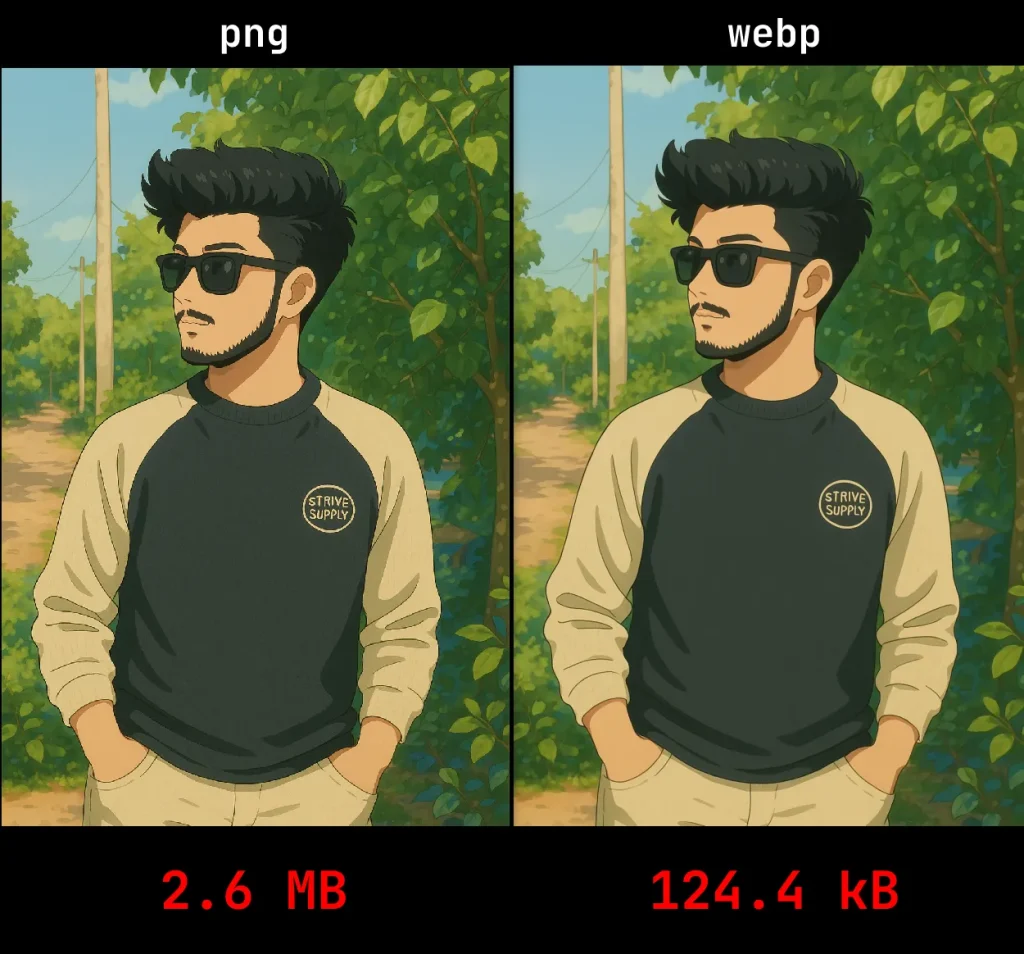
I converted my image to WebP, and both images show minimal difference, as it uses lossless compression. Before conversion, the image was 2.6MB, and after converting to WebP format, the size reduced to 124.6KB. This demonstrates an efficient way to compress images for website use.
so we need to compare other compression , i convert image into png
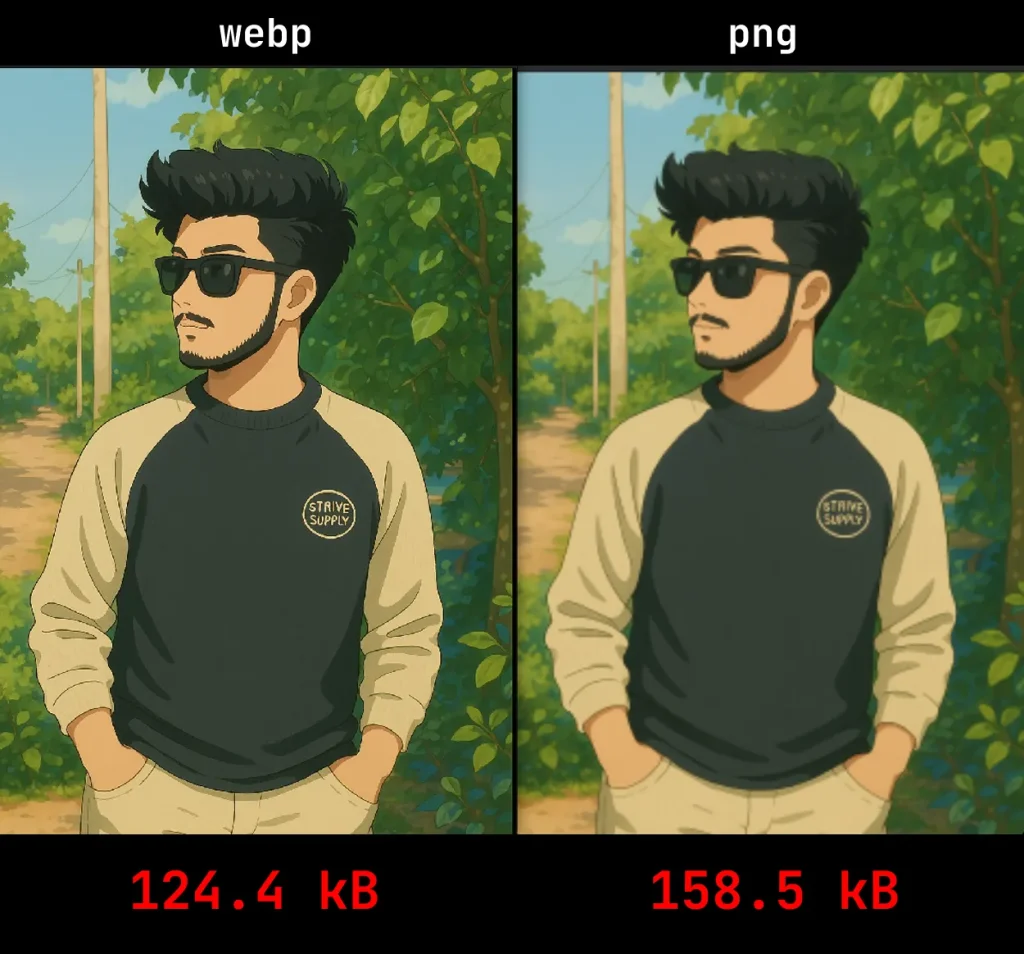
still png image size higher then webp but webp format quality is higher, i search in google “which image format is most suitable for web application? ” its give a results webp format, its low size and high quality then others
Why image size is importent for webapplication
Reducing image size directly improves image loading speed. For instance, imagine a user visiting your website, but the images are large in size, causing slow loading times. This delay can frustrate the user, and they might leave your site before it fully loads. As a result, this negatively impacts the overall user experience.
When the user experience is affected, it also has a detrimental effect on your website’s SEO. Search engines prioritize websites that offer a good user experience, including fast loading times. Slow websites tend to rank lower, which can lead to decreased visibility and traffic.
Lets go to Code
first of all create virtual envirement for it, in this purpose use this command line that create an virtual envirement.
python3 -m venv venv
then activate that virtual envirement , this purpose use this command
source venv/bin/activate
next we need to install some depentancies ,
pip install flask pillow werkzeug
in this project i create very simple file structure for this project, not complex , this is one page webapplication i just write css in this same html code.
image-to-webp-converter
├── app.py # This is for flask application
├── image_converter.py # Python module for convert img to webp
├── templates/ # HTML files
│ ├── index.html # Main landing page with the form
│
├── uploads/ # Directory for uploaded images
│ └── (images to be converted)
Python module for IMG to WEBp
from PIL import Image
import os
def convert_image_to_webp(input_image_path):
with Image.open(input_image_path) as img:
output_filename = os.path.splitext(os.path.basename(input_image_path))[0] + '.webp'
output_path = os.path.join(os.path.dirname(input_image_path), output_filename)
# Save the image as WebP
img.save(output_path, 'WEBP')
return output_filename
its a very simple module i use python library call pillow , in this PIL is (Python Imaging Library) it handles image files, and i import os , this is help to interact with file systems
i create function , that name is convert_image_to_webp()
and in this code Image.open(input_image_path) its help to locating input image file location
output_filename = os.path.splitext(os.path.basename(input_image_path))[0] + '.webp'
os.path.basename its get the name of the file, and os.path.splitext() this splitting the file’s name and file extension, [0] its define the file name , ‘.webp’ its added to create new file name.
output_path = os.path.join(os.path.dirname(input_image_path), output_filename)
os.path.dirname(input_image_path) this gets the folder of original image, and os.path.join()
combines this directory with the new file name photo.webp
to create the full path where the WebP image will be saved.
img.save(output_path, 'WEBP')
this line is save the image WEBP into output path
Python code for Create a Flask Application
from flask import Flask, render_template, request, send_file
import os
from werkzeug.utils import secure_filename
from image_converter import convert_image_to_webp
app = Flask(__name__)
# Set upload folder and allowed extensions
app.config['UPLOAD_FOLDER'] = 'uploads'
app.config['ALLOWED_EXTENSIONS'] = {'png', 'jpg', 'jpeg'}
# Ensure the upload folder exists
os.makedirs(app.config['UPLOAD_FOLDER'], exist_ok=True)
# Function to check allowed file extensions
def allowed_file(filename):
return '.' in filename and filename.rsplit('.', 1)[1].lower() in app.config['ALLOWED_EXTENSIONS']
@app.route('/')
def index():
return render_template('index.html')
@app.route('/upload', methods=['POST'])
def upload_image():
if 'file' not in request.files:
return 'No file part', 400
file = request.files['file']
if file.filename == '':
return 'No selected file', 400
if file and allowed_file(file.filename):
filename = secure_filename(file.filename)
file_path = os.path.join(app.config['UPLOAD_FOLDER'], filename)
file.save(file_path)
# Convert the uploaded image to WebP format
output_path = convert_image_to_webp(file_path)
return render_template('index.html', converted_image=output_path)
return 'File not allowed', 400
@app.route('/download/<filename>')
def download_file(filename):
return send_file(os.path.join(app.config['UPLOAD_FOLDER'], filename), as_attachment=True)
if __name__ == '__main__':
app.run(debug=True)
this code for create flask webapplication , after importing our module in this app.py we can use that module
from image_converter import convert_image_to_webp
next we need to HTML code for website interface , but i am not include that code in this blog , i will give link for this code
and thank you for reading my blog , and i will give source code for this project , if decited to lonch this application you have a one problem , you need to market this , you need to optimaize SEO for your site , now its very tuff compatition available for this kind of micro saas app.
i give suggestion for this , choose your micro niche and fix your target audiense and target Keywords to rank in google search (so write SEO friendly content), then only you can make consistently make passive income .
keep touch with me i will share my next saas ideas with source code
Explore Our GitHub Repository
Check out the code and collaborate on the image-to-webp-format-flask-application project!
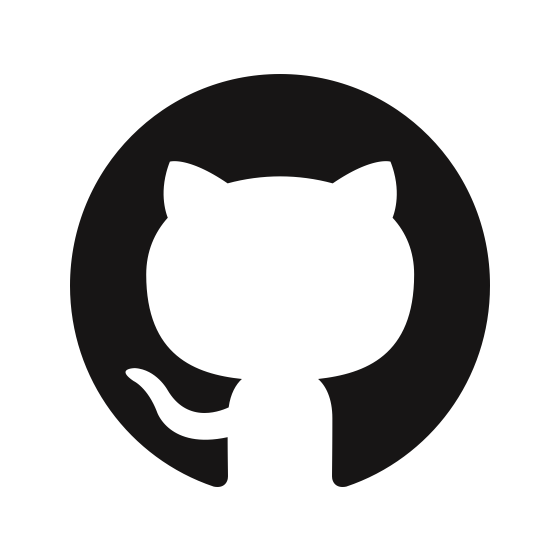