I am Aathil Ahamed; my nickname is Aathil Ducky. Currently, I am in an internship period. I decided to start my career in cybersecurity in my university’s final year. At that time, I was searching for cybersecurity projects; I did not get any ideas.
One day I was playing CTF, that time I got an idea, I decided to create a web application for sensitive information gathering (Sensitive information gathering with GUI interface)
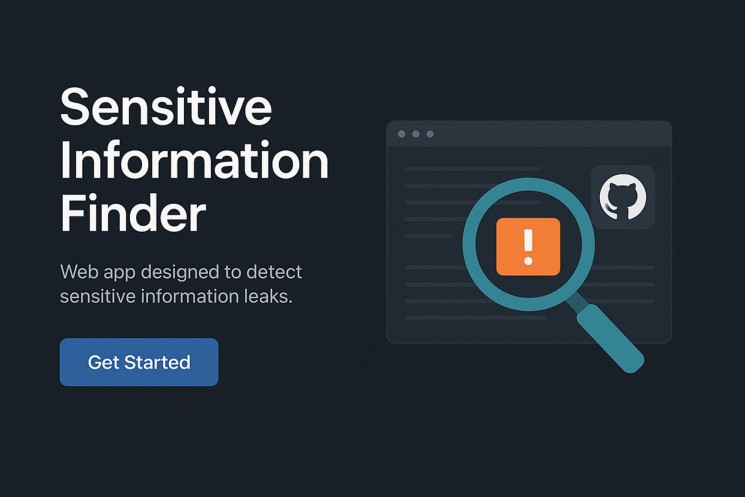
Key Features of a Cybersecurity Sensitive Info Gathering Tool
- Subdomain Gathering
- Directory enumeration
- google dorking
- Web crawling
- Web scraping
- Like finding all the website assets
- and it’s storing all the findings in sqlite database
- it has one of the functions when I give all the website assets links it scans all the resources, finding sensitive information like publicly available API keys, and sensitive information like passwords ..etc(i used regex pattern matching for this work)
- git repo scanning (when given a git repository link it temporarily downloads the repository, then scans the full repository its finding sensitive information leakages, with which line of code)
- all the senstive information findings stored in sqlite database
- Some features are available in this project, but I did not menstioned
this is my project
In this project show tables, we can see stored information and scan items etc, Each item is stored in different tables. in this project, I wrote Python modules for each functionality. I used the Django web development framework to make these tools in the graphical user interface.
But already tools are available for this purpose, but the key selling point of my project is the graphical user interface, and I include sqlite database, it can be maintain and reused the information.
when you use another tool we need to use it in a terminal interface. Sometimes it’s hard for non-technical people, that why i created this tool in a GUI interface. i include a feature when you domain name as a input its automatically gathers website assets
Source code & Resources
Explore Our GitHub Repository
Check out the code and collaborate on the duck-duck-leaks project!
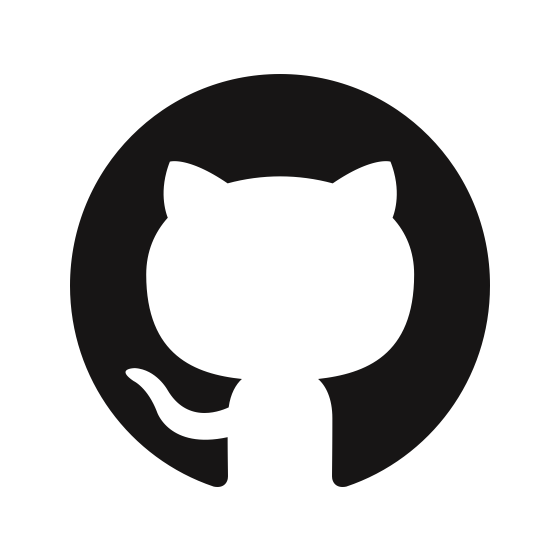
How I Scan Sensitive Information (Regex)
I use regex pattern matching for a sensitive information finder, Regex is a regular expression. use of regular expressions is for searching, matching, manipulating the strings. It’s widely used for manipulating strings and searching specific patterns within texts, validating the formats of strings, updating parts of the string ..etc
re.match(): Checks if the beginning of a string matches the regex pattern.
re.search(): Searches the entire string for the first occurrence of the pattern.
re.findall(): Returns a list of all non-overlapping matches in the string.
re.finditer(): Returns an iterator yielding match objects for all non-overlapping matches.
re.sub(): Replaces occurrences of the pattern in a string with a replacement.
i will give simple code for regex pattern matching
import re
# Pattern for finding email
pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}'
# some sample text
text = "Please contact us at info@aathilducky.com or aathilducky@gmail.com."
# Search for all email addresses in the text
matches = re.findall(pattern, text)
print(matches) # Output: ['info@aathilducky.com', 'aathilducky@gmail.com']
We can use other methods also for sensitive findings, like data analysis and others, but I did not use data analysis in my cybersecurity final year project because data analysis needs more computations and it’s more time-consuming than regex pattern matching
and the analysis method is that it has a low false positive compared to regex, but I still use regex pattern matching. I reduce false positives using optimizing the patterns
This is my module for regex pattern matching
import re
# Define your regex patterns for secrets
_regex = {
'google_api': r'AIza[0-9A-Za-z-_]{35}',
'google_oauth': r'ya29\.[0-9A-Za-z\-_]+',
'google_api_key': r"(?i)AIza[0-9A-Za-z\\-_]{35}",
'google_recaptcha_key': r"(?i)(\"|\')6[0-9a-zA-Z-_]{39}(\"|\')",
'generic_api_key_inurl': r'https?://[^\s]+[?&](key|api_key|apikey|apiKey|ApiKey|access_token|auth|authentication|token|secret|client_id|client_secret|API_KEY|private_key)=[a-zA-Z0-9_\-]+',
'generic_api_key_incode': r'\b(key|api_key|apikey|apiKey|ApiKey|access_token|auth|authentication|token|secret|client_id|client_secret|API_KEY|private_key)\s*=\s*["\']([a-zA-Z0-9_\-]+)["\']\s*;?',
'firebase' : r'AAAA[A-Za-z0-9_-]{7}:[A-Za-z0-9_-]{140}',
'firebase_api_key_pattern' : r'AIza[0-9A-Za-z-_]{35}',
'firebase_database_url_pattern' : r'https://[a-z0-9-]+\.firebaseio\.com',
'Firebase API Key': r"(?i)firebase_api_key[:=]\s*['\"]?([a-zA-Z0-9]{40})['\"]?",
'recaptcha_secret_key_pattern' : r'(?i)(?:=|\'|")?(6L[0-9A-Za-z]{39})(?:=|\'|")?',
# it will continue .......
}
def find_secrets(text):
findings = {}
for name, regex in _regex.items():
matches = re.findall(regex, text)
if matches:
findings[name] = matches
return findings
This result of regex scanning
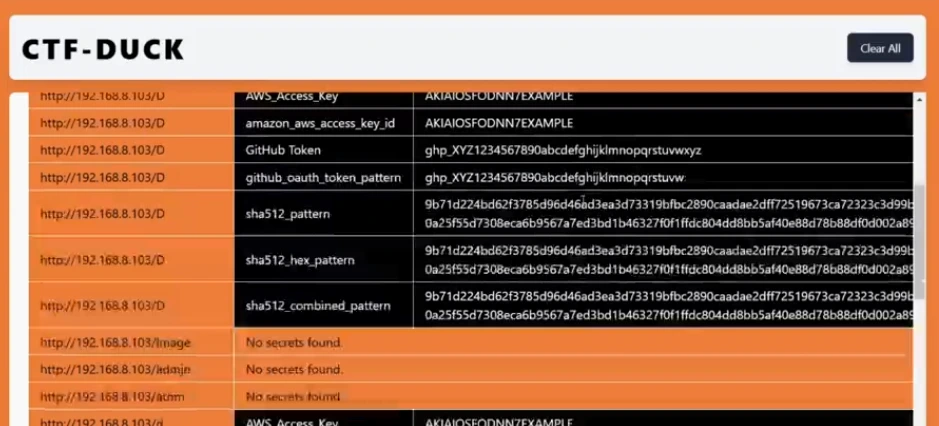
My project has a lot of functionalities, so I can’t explain everything, so I will explain a few things
Subdomain Finder
In this task, I have to find all the subdomains of the given domain name. in this task, i use requests, sublist3r libraries, I create a python module for this functionality. First of all, we have to install these libraries
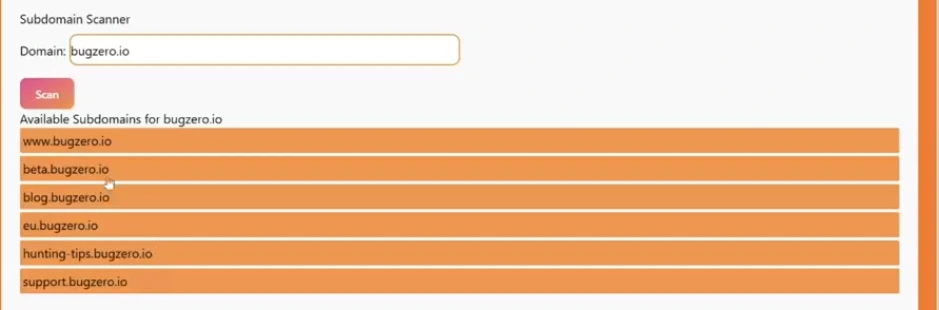
pip install requests sublist3r
this is my website module source code
import requests
import sublist3r
def gather_subdomains(domain):
# Gather subdomains using Sublist3r
subdomains = sublist3r.main(domain, 40, None, ports=None, silent=True, verbose=False, enable_bruteforce=False, engines=None)
available_subdomains = []
# Check each subdomain for availability
for subdomain in subdomains:
url_http = f"http://{subdomain}"
url_https = f"https://{subdomain}"
try:
# Try HTTPS first
response = requests.get(url_https, timeout=5)
protocol = 'https'
status_code = response.status_code
except requests.RequestException:
try:
# Fallback to HTTP if HTTPS fails
response = requests.get(url_http, timeout=5)
protocol = 'http'
status_code = response.status_code
except requests.RequestException:
# If both HTTP and HTTPS fail, skip the subdomain
continue
# Append as a tuple (subdomain, protocol, status_code)
available_subdomains.append((subdomain, protocol, status_code))
return available_subdomains
I can not give every source in this blog at the beginning of this blog, I embedded the GitHub repository.
Git Repo Scan
I’m going to explain this Git scan functionality
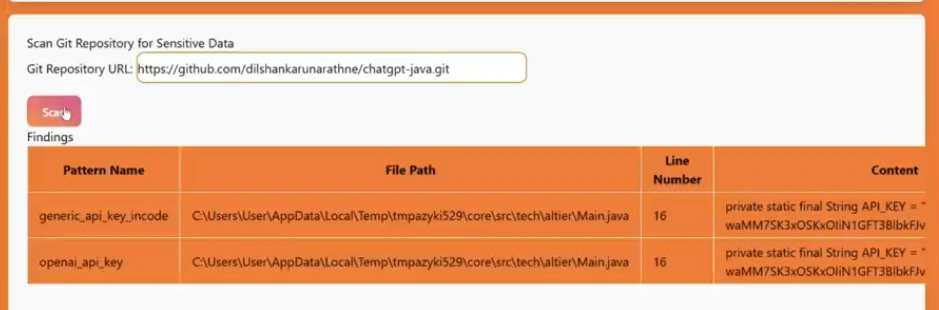
When I give a link in the input section, it downloads that git repository temporarily, then it scans that repository using the same regex pattern matching.
If it finds any sensitive information it shows the publicly available information, and it’s stored in a database, with which line of code that helps to find sensitive information’s the exact location
Link Scrapper
When I give a website link, it scrapes the website and gives the source code files. after that, we can use that to further process, like scanning the source code files for finding sensitive information.
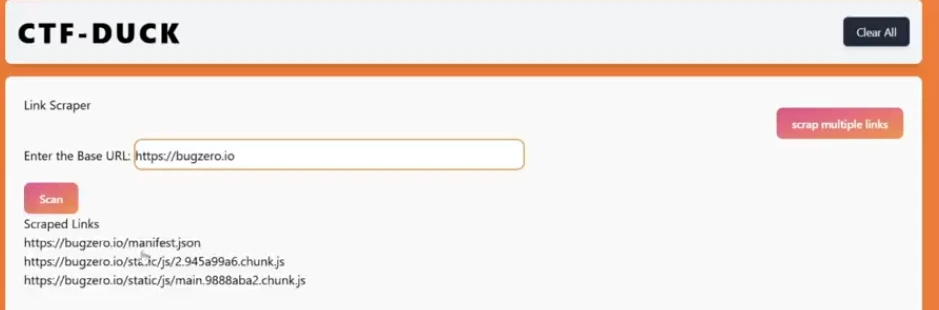
I write Python module for this functionality –> Linkscrapper.py
Directory Enumeration
i write python module for directory enumeration but i am not preferable for this functionality becouse gobuster , fuff , dirbuster ..etc like better tools available now so that tools are more faster then my project module, and my module is more time consuming then others, and but my tool is graphical user interface that is one advantage
if you want to see my code –> Directory enumeration module
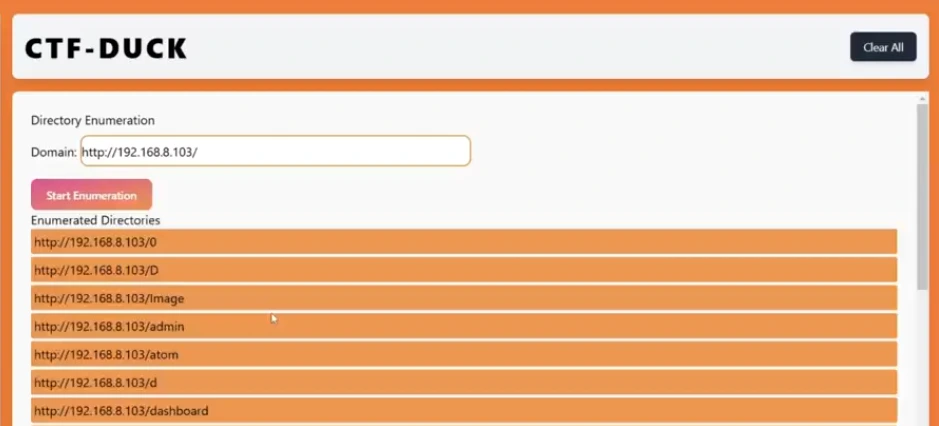
Google Dorks
my GUI web application have google dorks options also, how i create that, if i use google dorks i need google api so that i use google search but its have one disadvantage its only give top 10 results only.
and i create this like in the right corner have set of google dorks commands, i can just drag and drop that dork commands in input field after that when i press scan, its give some results
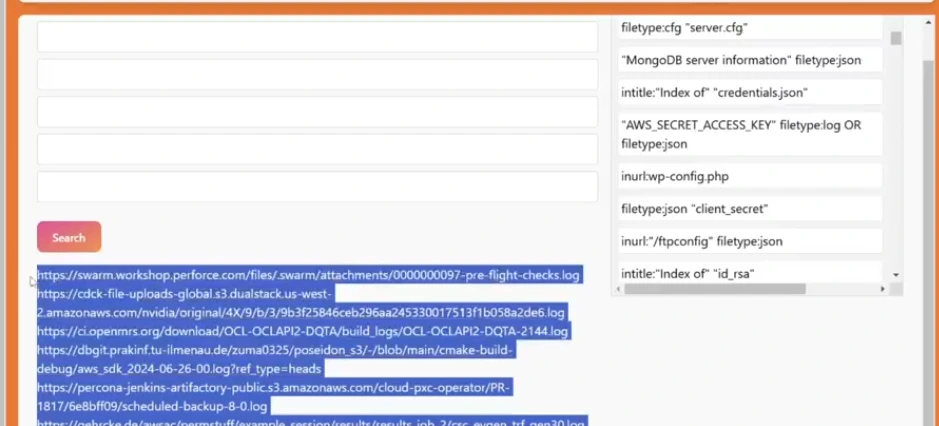
after that i can paste that links in to secret finder, and some time google dorks directly show sensitive informations, i collect this google dorks commands from hackerone bugbounty reports, and most common google dorks command from google, and exploit database (Google Hacking Database), chatgpt and more i collect from lot of resources
Finding any base64 encoded values
this also include in my project, this functionality works is when i give any links in this input section its scan the website if any base64 encoded values in this link it’s automaticaly decoded that base64 value.
once upon a time i was play CTF i think that is vulnhub mrrobot challage, i solved that CTF defferent method its take long time, i bruteforce my target and i put lot of work, but this CTF is very simple username and password give in this website but that are base64 encoded, that situation make me to build this module and i include this in to my final year cyber security project
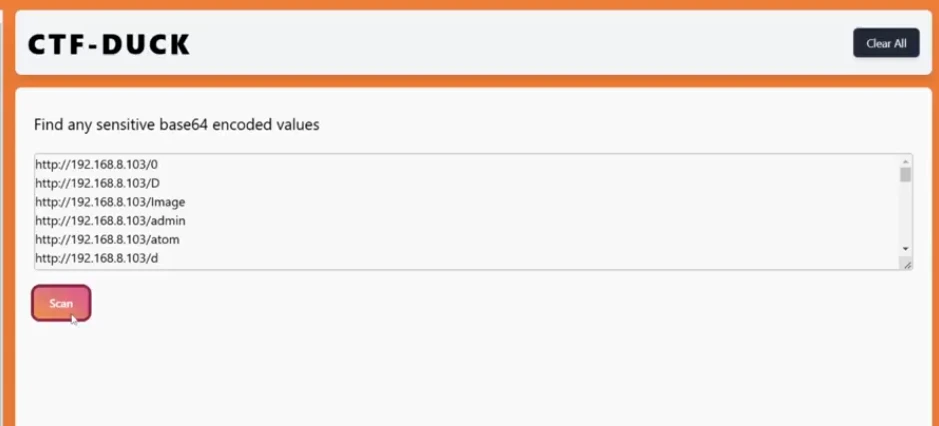
This project has been an exciting journey in the field of cybersecurity, combining practical tools like subdomain gathering, directory enumeration, and sensitive information scanning, all within a user-friendly GUI.
By utilizing regex pattern matching and integrating features like Git repo scanning, Google dorking, and base64 decoding, the project provides an easy-to-use solution for security researchers. Although similar tools exist, my focus on accessibility and reusability through the SQLite database sets this tool apart.
As I continue my internship and progress in cybersecurity, I look forward to refining and expanding these capabilities.
I am working on another cybersecurity project now. Keep connected with me, I will update
Pingback: Build a URL Shortener with Python, Flask & MySQL, Tailwindcss - AATHIL DUCKY
Pingback: Interactive Snake Game Using OpenCV & Hand Tracking - AATHIL DUCKY
Pingback: Build a Micro SaaS Image to WebP Converter Using Python Flask - AATHIL DUCKY
Pingback: Python code Text to Speech Tasks using Google Colab - AATHIL DUCKY